Python String Formatting and Tricks By albro
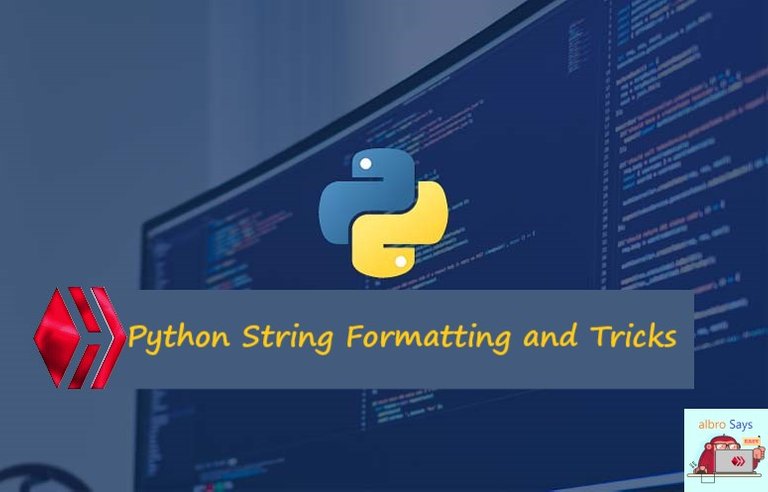
In different projects, we need to combine variables and text strings. With the help of string formatting in Python, we can create a regular structure to print the variable between the text string. These constructs help make the code more readable and of course improve debugging and development. In this post, I'll talk about 3 ways to format Python text strings.
We have two variables named name
and power
; The first one specifies the person's name and the second one specifies his power. Suppose the name of the person is albro
and his power is 90
, and we want to print a text similar to the following in the output:
albro power is: 90
We use the print()
function to print texts in the console. The first method is to use the print tricks in Python to connect the variable and the string and print it in the output. As a result, we have a code similar to the following:
name = "albro"
power = 90
print( name + "'s power is:", power )
If the number of variables increases or if we want to create a more complex text string, this method does not look very interesting! Therefore, we use string formatting methods.
String formatting methods in Python
In addition to the fact that using variables directly in the string isn't interesting, it can sometimes hurt the readability of the code and the development process! So always try to use the features of different languages to improve your programming skills.
There are three ways to format a string in Python:
- Using the
format()
method on the string - Using the
f
method and placing it before the string definition - Using the
%-formatting
method, which is a bit older.
Python string formatting with format()
The str.format()
method in Python is used to organize long strings with many variables. Of course, this does not mean that it cannot be used for a short string of one variable.
This method is called on text string variables. str
means string data type in Python. This method is used to prepare the string.
To use format()
, we must determine the position of the variables in the string with {}
(open and closed braces). Consider the same first example. In the final text string, we had two variables. So we change the string as follows:
txt = "{}'s power is: {}"
Now it is enough to call the format method on the structured string and give it the variables.
txt = "{}'s power is: {}".format( name, power )
Now, if we print the value of the txt
variable in the output, we will have the following result:
print(txt)
# albro's power is: 90
What we did was the simplest way to use string formatting in Python. In this case, the position of the variables is counted from the leftmost position in the text and its equivalent should be determined as the input of the format()
function.
Determining the index of the variable in the format method
In order to specify the index of each variable in the string, it's enough to specify the desired index inside the braces. The code below is exactly the same as the default formatted string structure without specifying the indices.
txt = "{0}'s power is: {1}".format( name, power )
In the following code, I moved the index of the variables and as you can see in the output, the order of calling and placing the variables in the string has been moved.
txt = "{1}'s power is: {0}".format( name, power )
print(txt)
# 90's power is: albro
Names for variables within the string
In addition to the index number, we can determine the position of the variables with the help of the desired names. Just like when formatting a text string with Python, we set a variable name for each position and set a value for those variables when calling the format()
method.
In the following code, I have first defined a Python list called data
to determine the values. We could use single variables, or other data structures, as before. Then I choose a name for each position and use them when calling format()
.
data = ["ocdb", 80]
txt = "{name}'s power is: {power}".format( name=data[0], power=data[1] )
# ocdb's power is: 80
Pay attention that when specifying the inputs of the string format function, we must specify the name of the desired location.
Format strings with f-string
This method is very similar to the previous method; That is, instead of variables in the text string, we use braces. This method was added from Python version 3.6.
To format the Python string with f, it is enough to put the letter f before the text string and put the main name of global variables directly inside {}
. For a better understanding, consider the following example:
name = "ocdb"
power = 80
txt = f"{name}'s power is: {power}"
print(txt)
# ocdb's power is: 80
The letter we place before the beginning of the text string can be a lowercase (f) or an uppercase (F); The result will make no difference.
Suppose we have a list of three dictionaries in Python. One person's name and power are written in each dictionary. With the help of a for
loop, we print the power of these three people in the output:
powers = [{'name': 'ocdb' , 'power': 80},
{'name': 'albro', 'power': 95},
{'name': 'ecency' , 'power': 90}]
for hiver in powers:
print( f"{hiver['name']}'s power is: {hiver['power']}" )
# Result:
# ocdb's power is: 80
# albro's power is: 95
# ecency's power is: 90
Python string formatting with %
The third method can be considered the oldest method for formatting the text structure in Python. Of course, this does not mean that this method is outdated or ineffective. This type of formatting is used in many projects.
For this, we must first determine the location and type of variables within the text. This is done with the help of the percent sign (%
) and a letter that indicates the type of variable value. After finishing the text string, we give the desired values to the string by putting %
.
Notice the structure defined in the following code:
name = "albro"
power = 90
txt = "%s's power is: %d" % (name, power)
print(txt)
# albro's power is: 90
In this code, two positions %s
and %d
are determined in the string, and after the end of the string (after the sign "
), we have given the values we want to format the text by inserting a %
.
If we want to define only one variable in the string, we don't need to use tuple to refer its value and we can initialize it after putting %
.
name = "albro"
msg = "Thanks for visiting %s hive blog!" % name
print(msg)
# Thanks for visiting albro hive blog!
To determine the variable type, we have three main types:
%s
: String values%d
: Integer values%f
: Decimal values
https://leofinance.io/threads/albro/re-leothreads-2sml4cum5
The rewards earned on this comment will go directly to the people ( albro ) sharing the post on LeoThreads,LikeTu,dBuzz.
Congratulations @albro! You have completed the following achievement on the Hive blockchain And have been rewarded with New badge(s)
Your next target is to reach 9000 upvotes.
You can view your badges on your board and compare yourself to others in the Ranking
If you no longer want to receive notifications, reply to this comment with the word
STOP
Check out our last posts:
Yay! 🤗
Your content has been boosted with Ecency Points, by @albro.
Use Ecency daily to boost your growth on platform!
Support Ecency
Vote for new Proposal
Delegate HP and earn more
As a Guest curator for Ecency, I think that you provided value with such explanations.
Congratulations!
✅ Good job. Your post has been appreciated and has received support from CHESS BROTHERS ♔ 💪
♟ We invite you to use our hashtag #chessbrothers and learn more about us.
♟♟ You can also reach us on our Discord server and promote your posts there.
♟♟♟ Consider joining our curation trail so we work as a team and you get rewards automatically.
♞♟ Check out our @chessbrotherspro account to learn about the curation process carried out daily by our team.
🏅 If you want to earn profits with your HP delegation and support our project, we invite you to join the Master Investor plan. Here you can learn how to do it.
Kindly
The CHESS BROTHERS team
Thanks for your contribution to the STEMsocial community. Feel free to join us on discord to get to know the rest of us!
Please consider delegating to the @stemsocial account (85% of the curation rewards are returned).
You may also include @stemsocial as a beneficiary of the rewards of this post to get a stronger support.