Python Time and Tricks By albro
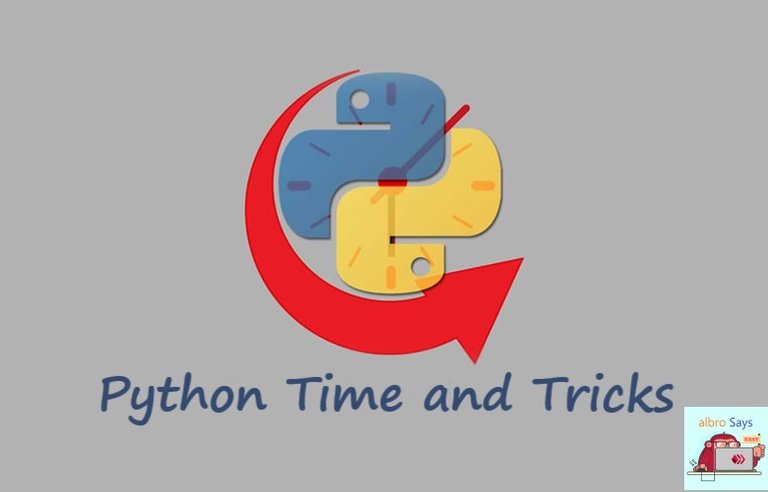
The element of time has various uses in the development of different programs. We may want to check when the request was sent. Or create an interruption during the execution of the program. In this post, I'll deal with working with time in Python. I use the time
library in Python and work with different functions.
Time in computer systems
As you may know, time in computer systems is measured in seconds. All times have a time origin. The origin of computer time is January 1, 1970 at 00:00 AM. The time in the computer is calculated as seconds that have passed since the original time. It can then be converted to hours and dates by special arithmetic conversion.
There are many modules and libraries for working with time in Python. They are mostly used for a specific application in programming.
One of the main modules provided by default with Python is the time
library.
This library provides us with various functions to get the current time of the system, calculate times and create interrupts.
Next, I will examine working with the time
module in Python.
time
library for working with time in Python
To use this module, we must first enter it into the program.
import time
Now I can do things related to time by calling different functions on time
.
Get system time
The first and one of the most used functions in this module is the time()
function. This function outputs the current system time. The output is in the form of a float
and it is the elapsed seconds from the time origin.
time.time()

Current time with today's date
There is another function in Python's time
module called localtime()
. This function returns an object of type time.struct_time
. This object has properties with the following name and information.
time.localtime()
# time.struct_time(tm_year=2023, tm_mon=5, tm_mday=19, tm_hour=12,
# tm_min=14, tm_sec=26, tm_wday=4, tm_yday=139, tm_isdst=1)
The properties of this object are:
tm_year
: Yeartm_mon
: Current monthtm_mday
: number of the day of the month (specifying the number of the monthtm_hour
: Current hourtm_min
: minute of current timetm_sec
: seconds of timetm_wday
: counting the day of the week (the most recent day of the current week, including Monday, equal to 0)tm_yday
: counting the day of the year (how many days of the year is today?)tm_isdst
: specifying whether daylight saving time is active
We can store this object in a variable and use its properties. For example, in the code below, I have printed the year and the day counter of the year.
now = time.localtime()
print( now.tm_year) # 2023
print( now.tm_yday) # 139

Convert seconds to hours and date of the day
We saw that with the help of the time()
function, we can get and keep the time in seconds. Now we may have a numerical value (seconds) that we want to convert to time.
To convert seconds to days and hours and minutes in Python, we will use the ctime()
function in the Python time library.
If we don't give it any value as input to the function, it will output the current time by default.
time.time()
# 1684483432.2221847
time.ctime()
# 'Fri May 19 12:33:54 2023'
time.ctime(1684483432.2221847)
# 'Fri May 19 12:33:52 2023'

Convert specific time and date to seconds
Suppose we have a specific date and time and want to calculate its second equivalent. For this, we will use the mktime()
function.
This function takes a struct_time object as input. (Its structure is exactly the same as the output structure of the localtime()
function.)
import time
t = time.localtime()
time.mktime(t)
# 1684483966.0
Basically, this function works the opposite of the localtime()
function.

Print time with special time format in Python
Most of the time, when working with time in Python, we need to display a time in a specific format. For this, you can use the strftime()
function.
This function has two inputs.
- The first input is a string specifying our desired format.
- The second input is an object of the
struct_time
class that specifies the time we want.
current_time = time.localtime()
time_string = time.strftime( "%m/%d/%Y %H:%M:%S", current_time )
print(time_string)
# 05/19/2023 12:49:17

Convert time in seconds format
If we want to do the opposite of the previous operation, we will use the strptime()
function. This function takes two inputs from us.
- The first input is a string specifying the input time format.
- The second input is the string and time we want.
time_string = "19 May 2023"
result = time.strptime( time_string, "%d %b %Y" )
print(result)
# time.struct_time(tm_year=2023, tm_mon=5, tm_mday=19,
# tm_hour=0, tm_min=0, tm_sec=0,
# tm_wday=4, tm_yday=139, tm_isdst=-1)
If the hours are not specified in the entered value, they are assumed to be zero.
Creating an interruption with the sleep
function
Sometimes in the development of programs, we need to stop the program for a certain period of time. For example, a task must be done 45 seconds after the completion of another activity.
Or in the programming of web crawlers to avoid blocking the activity of the robot, it is better to randomly interrupt the crawler.
When you communicate with the $Hive blockchain, you must create a certain interrupt between each comment for actions such as sending comments. Or when you want to trade, you need to have an interruption after the value of each token!
To interrupt the running program, the sleep()
function is used in the time
module.
The input of this function is a number that specifies the amount of seconds to interrupt the execution.
time.sleep( 45 )
Definition of random delay in Python
In order to define the program execution delay (interruption) randomly, you can easily use a Random
value.
The following code takes a random 1-2 minute break each time the Python loop executes.
import time
import random
while True:
# do something here
time.sleep( random.randint( 60, 120 ) )
Calculation of program execution time in Python
One of the most common uses of time tricks in Python by programmers is to calculate the execution time of the program.
With a simple trick and using the time
module in Python, we will be able to accurately calculate the duration of the code execution.
For this, we must register the current time using the time()
function before starting the desired piece of code and do the same after execution.
The difference between these two times will be equal to the program execution time.
For example, suppose we want to get the execution time of the following program.
In this simple program, we add the numbers 1 to 500 together with a for
loop and print them in the output.
sum = 0
for n in range(1,500):
sum += n
print(sum)
To calculate the execution time, before starting the for
loop, we put the current time in the start
variable by calling the time()
function.
At the end, by calling the same function again, we subtract the start time from it and display it in the output.
import time
start = time.time()
sum = 0
for n in range(1,500):
sum += n
print(sum)
print("Run Time: " + str( time.time() - start ))
The program execution time for me was about 0.022 seconds!
124750
Run Time: 0.02178672218322754
So easily we were able to calculate the execution time of the program in Python using the tricks of working with time that we learned.
Summary: time module
In this post, I talked about the time library in the Python programming language. Using its various functions, we took the current time of the system and converted different times to each other.
At first, we learned about the use of the time()
function, which outputs the current time of the system in the form of elapsed seconds. Then I converted this value to a readable format using the strftime()
function.
Next, I interrupted the execution of my program with the sleep()
function.
What techniques do you use to work with time in Python? Which function or method is empty in this post? The comments section is for your comments and questions.
Congratulations @albro! You have completed the following achievement on the Hive blockchain And have been rewarded with New badge(s)
Your next target is to reach 100 upvotes.
You can view your badges on your board and compare yourself to others in the Ranking
If you no longer want to receive notifications, reply to this comment with the word
STOP
To support your work, I also upvoted your post!
Check out our last posts:
Support the HiveBuzz project. Vote for our proposal!
https://leofinance.io/threads/view/albro/re-leothreads-xasggqry
The rewards earned on this comment will go directly to the people ( albro ) sharing the post on LeoThreads,LikeTu,dBuzz.
Can you demonstrate how you interact with the hive blockchain using python, thanks
Step by step soon❤️
Yay! 🤗
Your content has been boosted with Ecency Points, by @albro.
Use Ecency daily to boost your growth on platform!
Support Ecency
Vote for new Proposal
Delegate HP and earn more
Congratulations!
✅ Good job. Your post has been appreciated and has received support from CHESS BROTHERS ♔ 💪
♟ We invite you to use our hashtag #chessbrothers and learn more about us.
♟♟ You can also reach us on our Discord server and promote your posts there.
♟♟♟ Consider joining our curation trail so we work as a team and you get rewards automatically.
♞♟ Check out our @chessbrotherspro account to learn about the curation process carried out daily by our team.
🥇 If you want to earn profits with your HP delegation and support our project, we invite you to join the Master Investor plan. Here you can learn how to do it.
Kindly
The CHESS BROTHERS team
Thanks for your contribution to the STEMsocial community. Feel free to join us on discord to get to know the rest of us!
Please consider delegating to the @stemsocial account (85% of the curation rewards are returned).
You may also include @stemsocial as a beneficiary of the rewards of this post to get a stronger support.