Testing our knowledge of OOP / Probando nuestro conocimiento de OOP - Coding Basics #15
Putting It all to practice
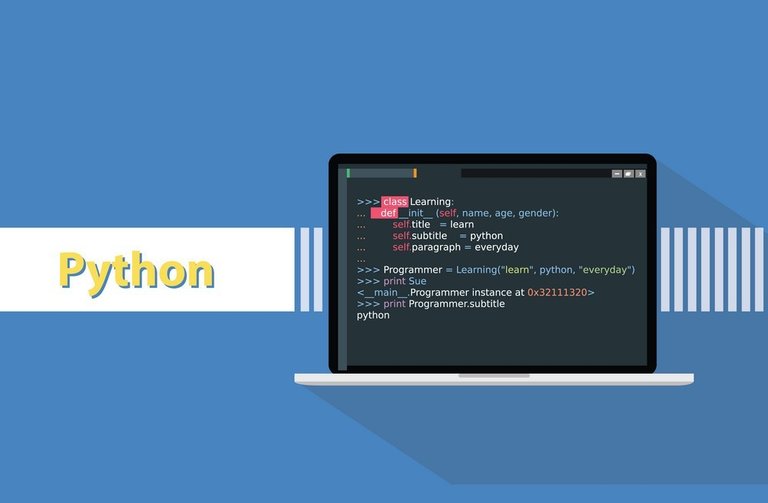
Shoutout to Robinson Muiru and LinkedIn
In this article you'll find:
- Introduction
- The problem
- Solution | Part I: Defining the Class
- Solution | Part II: Defining the Method in Child Classes
- Solution | Part III: The Cycle
- Solution | Part IV: Increasing the Complexity
Greetings to all!
In this edition of Coding Basics we will put into practice the knowledge acquired in OOP so far. In this case, we will use inheritance, polymorphism, encapsulation and abstraction.
If you want to test the acquired skills or learn something new, this is the perfect post for you. Just keep reading. That said.
The Problem
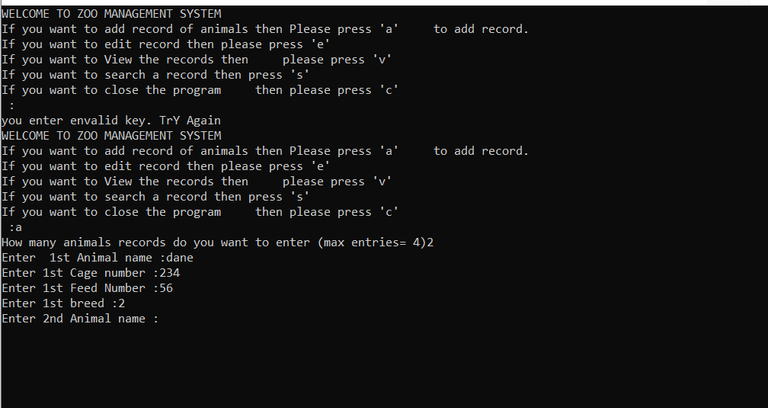
Shoutout to Code-Projects
You are a zookeeper who must feed the animals. Among them are the Lion, the Panda and the snake. Each of these species eats something different:
- The Lion eats meat
- The panda eats Bamboo
- The snake eats mice.
Reason why you must develop a system to feed each one. The specific requirements will be:
- You must use classes
- You must use a cycle to feed them
- You can only use one method for all animals.
This means that if we try to do this:
class Lion():
def feed_Lion():
print("Feeding Lion with Meat")
def feed_Panda():
print("Feeding Panda with Bamboo")
def feed_Snake():
print("Feeding Snake with Mice")
It will not be the correct option. This is why we will make use of certain tools learned in the previous Coding Basics to fulfill the conditions.
If you believe you can do it, take your time and solve the problem on your own.
If you want to learn how to do it, let's continue!
Solution | Part I: Defining the Class
Based on the concepts acquired during previous editions of Coding Basics, we must comply with the fact that we will always have to use a single method name to feed all the animals. What were those concepts that allowed us to use and overwrite methods in different classes?
- Polymorphism.
- Abstraction.
Thus, to create a method that can be used by all classes, the most sensible thing to do is to create a parent class (more about this when we talk about inheritance), which contains an abstract method called feed.
from abc import ABC, abstractmethod
class Animal(ABC):
@abstractmethod
def feed(self, food):
pass
Note: Remember that to use abstraction in Python, we must import from the abc library the ABC class and the abstractmethod decorator. In the same way, ABC must be placed as parent class.
Solution| Part II: Defining the method in the child classes
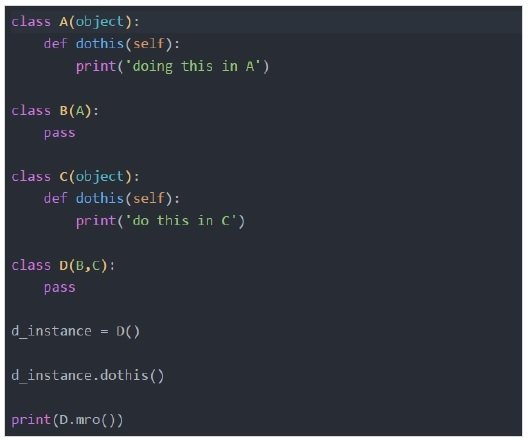
Shoutout to Code-Projects
Now, remembering that the feed method must be used by each of the classes representing animals, we use inheritance to create derived or child classes of Animal. Thus:
from abc import ABC, abstractmethod
class Animal(ABC):
@abstractmethod
def feed(self, food):
pass
class Panda(Animal):
class Lion(Animal):
class Snake(Animal):
But, how do we adapt the Feed function to each class? Simple.
With polymorphism, we know that when writing the same method in child classes, the parent method will be overwritten and the instructions will become those defined by the user in that class.
So, we just need to apply what we want to write.
from abc import ABC, abstractmethod
class Animal(ABC):
@abstractmethod
def feed(self, food):
pass
class Panda(Animal):
def feed(self):
print("Feeding the Panda with Bamboo")
class Lion(Animal):
def feed(self):
print("Feeding the Lion with Meat")
class Snake(Animal):
def feed(self):
print("Feeding the Snake with Mice")
Solution | Part III: The Cycle
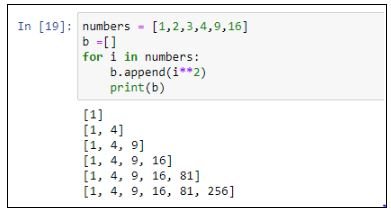
Shoutout to Simplilearn.com
This is the easiest part, where we only have to remember the use of for-cycles and lists.
If we want to use a for loop for different objects, we first have to create them. In this case, since each animal will be an individual class, we must create three of these.
However, if we recall, we know that for works best with iterable elements, which are collections of objects or variables in a single element. Thus, we use a list to create an object of each class.
zoo = [Lion(), Panda(), Snake()]
Thus, we will have an object to go through the list with the loop.
Now, when we run the loop:
for animal in zoo:
animal.feed()
Just by applying this, we are telling the for to go through each element of the list and execute the corresponding feed method. If we execute, we will have this:
>>>
Feeding the Panda with Bamboo
Feeding the Lion with Meat
Feeding the Snake with Mice
Thus, we will already have a system to feed the zoo.
If it was simple, why not make it a little more complex? How about, if by means of conditionals and parameters in our feed method we can give the user the option to feed the animals?
Solution | Part IV: Increasing the Complexity
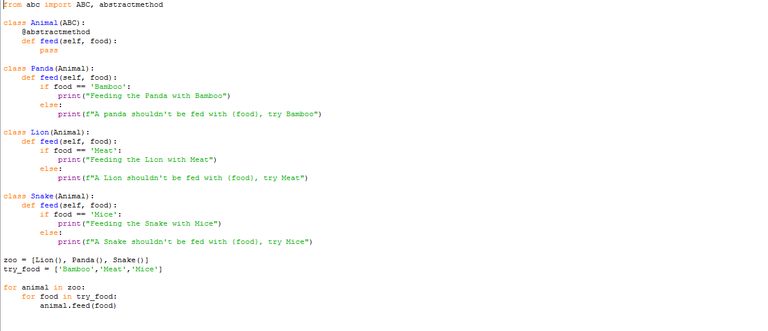
To make the system more efficient, we will again make use of abstraction, where we will add parameters to our already created abstract method. So, just add a parameter called food to each feed and use f-strings to apply it to each class:
class Animal(ABC):
@abstractmethod
def feed(self, food):
pass
class Panda(Animal):
def feed(self, food):
print(f "Feeding the Panda with {food}")
class Lion(Animal):
def feed(self, food):
print(f "Feeding the Lion with {food}")
class Snake(Animal):
def feed(self, food):
print(f "Feeding the Snake with {food}")
This will be useful in case we want to add parameters individually, but if we run it in a loop, we will have to create another list and another for loop for it to run.
zoo = [Lion(), Panda(), Snake()].
try_food = ['Bamboo','Meat','Mice']
for animal in zoo:
for food in try_food:
animal.feed(food)
However, if we do this, by having nested cycles, each animal will be fed all the elements of try_food. Because each animal has a different diet, we don't want this.
Therefore, we will use conditionals within the methods to verify that the correct option is being fed to the animals:
class Panda(Animal):
def feed(self, food):
if food == 'Bamboo':
print("Feeding the Panda with Bamboo").
else:
print(f "A panda shouldn't be fed with {food}, try Bamboo")
class Lion(Animal):
def feed(self, food):
if food == 'Meat':
print("Feeding the Lion with Meat").
else:
print(f "A Lion shouldn't be fed with {food}, try Meat")
class Snake(Animal):
def feed(self, food):
if food == 'Mice':
print("Feeding the Snake with Mice").
else:
print(f "A Snake shouldn't be fed with {food}, try Mice.")
Here, if we see for example the Panda, only when it is fed with Bamboo it will return that it is fed with bamboo. Otherwise, it will tell us that it cannot be fed with that type of food, and will ask us to use Bamboo. This will be repeated for each animal, and so, when we run: We will have the following:
A Lion shouldn't be fed with Bamboo, try Meat
Feeding the Lion with Meat
A Lion shouldn't be fed with Mice, try Meat
Feeding the Panda with Bamboo
A panda shouldn't be fed with Meat, try Bamboo
A panda shouldn't be fed with Mice, try Bamboo
A Snake shouldn't be fed with Bamboo, try Mice
A Snake shouldn't be fed with Meat, try Mice
Feeding the Snake with Mice
The best way to understand programming is through examples, and I hope that with this one you have been able to increase your understanding on the subject of object oriented programming.
If you want more exercises to reinforce the knowledge let me know.
Poniéndolo todo en práctica
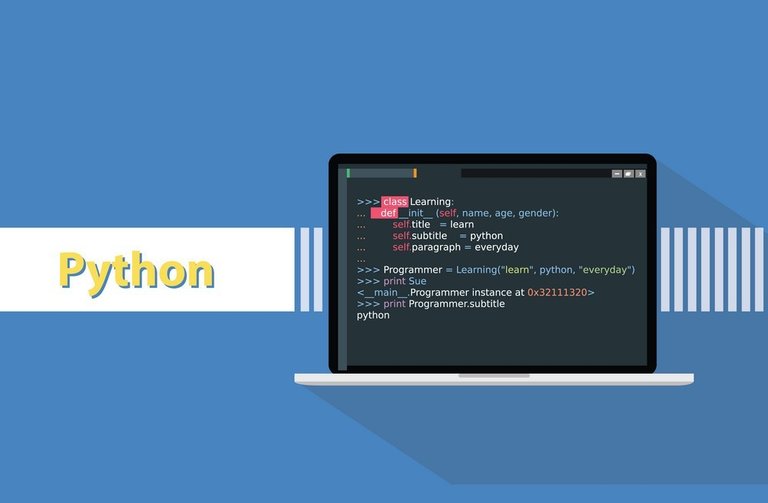
Shoutout to Robinson Muiru and LinkedIn
In this article you'll find:
- Introducción
- El problema
- Solución| Parte I: Definiendo la clase
- Solución| Parte II: Definiendo el método en las clases hijas
- Solución| Parte III: El ciclo
- Solución| Parte IV: Aumentando la complejidad
¡Un saludo a todos!
En esta edición de Coding Basics pondremos en práctica los conocimientos adquiridos en OOP hasta ahora. En este caso, usaremos herencia, polimorfismo, encapsulamiento y abstracción.
Si quieres poner a prueba las habilidades adquiridas o aprender algo nuevo, este es el post perfecto para ti. Solo tienes que seguir leyendo. Dicho esto.
El problema
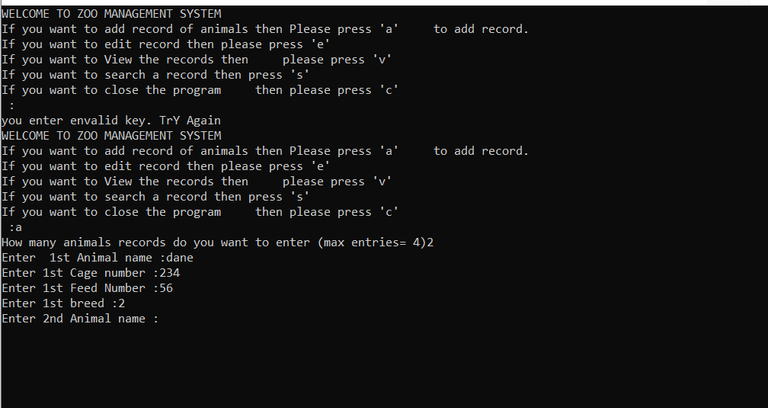
Shoutout to Code-Projects
Eres un encargado del zoológico que debe de alimentar a los animales. Entre estos están el Leon, el Panda y la serpiente. Cada una de estas especies come algo distinto:
- El Leon come carne
- El panda come Bamboo
- La serpiente come ratones.
Razón por la que debes de desarrollar un sistema para alimentar a cada uno. Los requerimientos específicos serán:
- Debes usar clases
- Deber usar un ciclo para alimentarlos
- Solo puedes usar un método para todos los animales.
Esto significa que si tratamos de hacer esto:
class Lion():
def feed_Lion():
print("Feeding Lion with Meat")
def feed_Panda():
print("Feeding Panda with Bamboo")
def feed_Snake():
print("Feeding Snake with Mice")
No será la opción correcta. Es por esto que nos valdremos del uso de ciertas herramientas aprendidas en los Coding Basics previos para cumplir con las condiciones.
Si piensas que puedes hacerlo, toma tu tiempo y soluciona el problema por tu cuenta.
Si quieres aprender como hacerlo. ¡Continuemos!
Solución| Parte I: Definiendo la clase
Basándonos en los conceptos adquiridos durante ediciones previas de Coding Basics, debemos de cumplir con que siempre tendremos que usar un solo nombre de método para alimentar a todos los animales. ¿Cuales eran aquellos conceptos que nos permitían usar y sobreescribir métodos en distintas clases?
- El polimorfismo.
- La abstracción.
De esta forma, para crear un método que pueda ser usado por todas las clases, lo más sensato será crear una clase padre (Más sobre esto cuando hablamos de herencia), que contenga un método abstracto llamado alimentar o feed.
from abc import ABC, abstractmethod
class Animal(ABC):
@abstractmethod
def feed(self, food):
pass
Nota: Recordamos que para usar abstracción en Python, debemos de importar de la librería abc la clase ABC y el decorador abstractmethod. De igual forma, se debe colocar como clase padre a ABC.
Solución| Parte II: Definiendo el método en las clases hijas
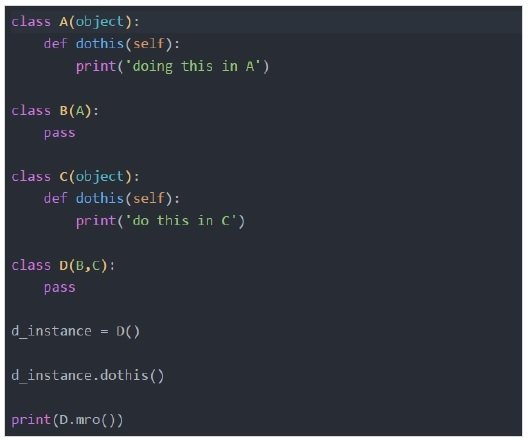
Shoutout to Code-Projects
Ahora, recordando que el método feed debe ser usado por cada una de las clases que represente los animales, usamos la herencia para crear clases derivadas o hijas de Animal. Así:
from abc import ABC, abstractmethod
class Animal(ABC):
@abstractmethod
def feed(self, food):
pass
class Panda(Animal):
class Lion(Animal):
class Snake(Animal):
Pero, ¿Cómo adecuamos la función Feed a cada clase? Simple.
Con polimorfismo, sabemos que al escribir el mismo método en clases hijas, el método padre se sobreescribirá y las instrucciones pasarán a ser las definidas por el usuario en esa clase.
Así, solo basta con aplicar lo que queremos escribir.
from abc import ABC, abstractmethod
class Animal(ABC):
@abstractmethod
def feed(self, food):
pass
class Panda(Animal):
def feed(self):
print("Feeding the Panda with Bamboo")
class Lion(Animal):
def feed(self):
print("Feeding the Lion with Meat")
class Snake(Animal):
def feed(self):
print("Feeding the Snake with Mice")
Solución| Parte III: El ciclo
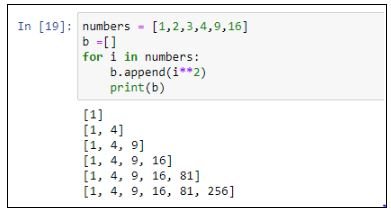
Shoutout to Simplilearn.com
Esta es la parte más sencilla, donde solo tenemos que recordar el uso de los ciclos for y de las listas.
Si queremos usar un ciclo for para distintos objetos, primero tenemos que crearlos. En este caso, ya que cada animal será una clase individual, debemos de crear tres de estos.
Sin embargo, si recordamos, sabemos que for trabaja mejor con elementos iterables, que son colecciones de objetos o variables en un solo elemento. Así, empleamos una lista para crear un objeto de cada clase.
zoo = [Lion(), Panda(), Snake()]
Así, tendremos un objeto por el que pasar en la lista con el ciclo.
Ahora, cuando ejecutemos el ciclo:
for animal in zoo:
animal.feed()
Con solo aplicar esto, le estamos diciendo al for que pase por cada elemento de la lista y ejecute el método feed correspondiente. Si ejecutamos, tendremos esto:
>>>
Feeding the Panda with Bamboo
Feeding the Lion with Meat
Feeding the Snake with Mice
Así, ya tendremos un sistema para alimentar al zoológico.
Si se te hizo sencillo, ¿Por qué no hacerlo un poco más complejo? ¿Que tal, si por medio de condicionales y parámetros en nuestro método feed podemos darle al usuario la opción de alimentar a los animales?
Solución| Parte IV: Aumentando la complejidad
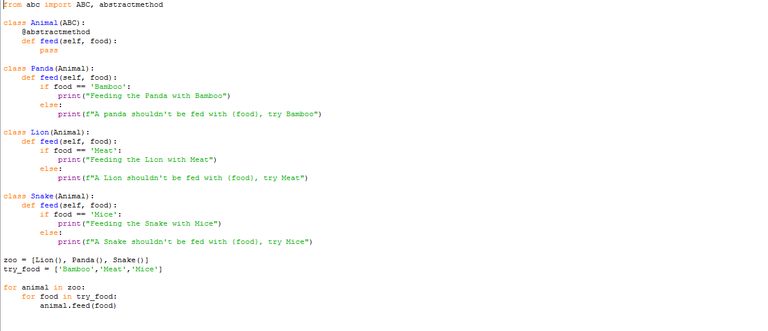
Para hacer el sistema más eficiente, nos valdremos de nuevo del uso de la abstracción, donde añadiremos parámetros a nuestro método abstracto ya creado. Así, solo basta con añadir un parámetro llamado food a cada feed y usar f-strings para aplicarlo a cada clase:
class Animal(ABC):
@abstractmethod
def feed(self, food):
pass
class Panda(Animal):
def feed(self, food):
print(f"Feeding the Panda with {food}")
class Lion(Animal):
def feed(self, food):
print(f"Feeding the Lion with {food}")
class Snake(Animal):
def feed(self, food):
print(f"Feeding the Snake with {food}")
Esto nos servirá en caso de que querramos añadir parámetros individualmente, pero si lo ejecutamos en un ciclo, tendremos que crear otra lista y otro ciclo for para que se ejecute.
zoo = [Lion(), Panda(), Snake()]
try_food = ['Bamboo','Meat','Mice']
for animal in zoo:
for food in try_food:
animal.feed(food)
Sin embargo, si llevamos a cabo esto, al tener ciclos anidados, se alimentará a cada animal con todos los elementos de try_food. Debido a que cada animal tiene una dieta distinta, no queremos esto.
Es por eso, usaremos condicionales dentro de los métodos para verificar que se esté alimentando a los animales con la opción correcta:
class Panda(Animal):
def feed(self, food):
if food == 'Bamboo':
print("Feeding the Panda with Bamboo")
else:
print(f"A panda shouldn't be fed with {food}, try Bamboo")
class Lion(Animal):
def feed(self, food):
if food == 'Meat':
print("Feeding the Lion with Meat")
else:
print(f"A Lion shouldn't be fed with {food}, try Meat")
class Snake(Animal):
def feed(self, food):
if food == 'Mice':
print("Feeding the Snake with Mice")
else:
print(f"A Snake shouldn't be fed with {food}, try Mice")
Aquí, si vemos por ejemplo al Panda, solo cuando se alimente con Bamboo nos retornará que se alimenta con bamboo. En caso contrario, nos dirá que no se puede alimentar con ese tipo de alimento, y nos pedirá que usemos Bamboo. Esto se repetirá para cada animal, y así, cuando ejecutemos: Tendremos lo siguiente:
A Lion shouldn't be fed with Bamboo, try Meat
Feeding the Lion with Meat
A Lion shouldn't be fed with Mice, try Meat
Feeding the Panda with Bamboo
A panda shouldn't be fed with Meat, try Bamboo
A panda shouldn't be fed with Mice, try Bamboo
A Snake shouldn't be fed with Bamboo, try Mice
A Snake shouldn't be fed with Meat, try Mice
Feeding the Snake with Mice
La mejor forma de entender la programación es por medio de ejemplos, y espero que con este hayas podido aumentar tu comprensión sobre el tema de la programación orientada a objetos.
Si deseas más ejercicios para reforzar el conocimiento dejamelo saber.
Thanks for your contribution to the STEMsocial community. Feel free to join us on discord to get to know the rest of us!
Please consider delegating to the @stemsocial account (85% of the curation rewards are returned).
You may also include @stemsocial as a beneficiary of the rewards of this post to get a stronger support.