App Development Log: Re-Creating The Windows Explorer Icon
WARNING: This is a technical programming article and is quite capable of boring you to tears and/or sending you into a coma. You have been warned.
At work, I am writing quite a chunky Windows Forms application. It's starting to get out of hand, and my inadequacies with GitHub and version control are hardly helping.
Due to privacy and security concerns, I can't share much of it, besides the necessary revealing of a couple of the remote devices I communicate with which contain generic names.
...'everyone uses that same bloody Visual Studio Code picture. As I use this editor myself I thought I'd create my own, but with fingerprints and dust included'...
I am currently working in the medical arena and these devices are prefixed 'VD' for venereal disease. It fits perfectly as my application will ultimately aid people who shag around a lot and get the clap often.
These computers are riddled with the likes of Syphilis, Gonorrhea, Chlamydia (aka 'The Clap'), and many other lovely diseases designed to irritate your groin areas and cause you to scratch fervently all day.
What I wanted was the perfect Windows Explorer icon; the remote version with that green pipe. After some digging, I discovered this icon to be in the file ‘C:\Windows\System32\imageres.dll’
I found it easily the first time around. This time I was getting eyestrain, many icons look the same and all I can say is this resource file is big.
Finding it is the first challenge, but you then need to extract it and guess at the correct size. These range from 16x16 pixels to 320x320 on occasion. You might think it may be best to grab the largest size and then scale it down and yes, I tried this.
The result was an icon that didn’t quite have the correct shading. I was being a pedantic bastard and wanting to find a cure for Syphilis faster, it needed to look good. My balls were starting to itch thinking about it all.
I settled on 48x48 after sizing up the icon with what I could see in Windows Explorer. It looked about right and that was the first step.
Next was the font. I tried ChatGpt and asked it what font Microsoft uses for their Windows Explorer icon textual description. It told me they do not disclose those facts but it's probably Segoe UI, 9pt. Very fucking useful.
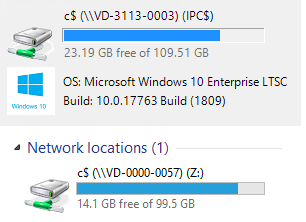
...'if you have not guessed already, my iteration is the top one. Close but not perfect'...
That's the default VB.Net font and it looked similar, so far so good. My font looks a little more spaced and a little less bold. Adding Bold made it over the top, that's not the answer.
I figured it was a grey background versus a white background that caused theirs to stand out more.
The font on the statistics looked the same, but the colour was greyer. Guessing their colours is trial and error. I tried a few and settled on simple 'GrayText'
Next was that thing that looks like a progress bar. Why not use the default Progress Bar Control? It seemed to do the job well and besides some stupid spacing limitations on the top edge, it looks almost the same.
The colour of it was the next problem. No amount of googling was telling me what it was. I settled on Dodger Blue, though I am not convinced. Mine looks a little brighter than the real thing.
frm_main.prg_drivespace.ForeColor = Color.DodgerBlue
If you're going to display the icon then you need some code to do the donkey work. As the Remote Registry Service is disabled at work, I had to rely on what was enabled, namely PS Remoting.
If you don't know what this is, then it gives you the ability to run Powershell scripts as if they were running on a remote device. Powerful stuff and something I have not had the privilege to use until now.
Powershell is shit for forms as it's a shell scripting language, so I designed the User Interface in VB.Net Forms, intending to shell out to Powershell for all the dirty work.
Function GetDriveSpace(ScriptLocation As String, Script As String, Device As String) As Boolean
' Calls the Powershell script, 'GetDriveSpace.ps1'
Dim ScriptPath As String = ScriptLocation & "\" & Script
Dim StartInfo As New ProcessStartInfo()
StartInfo.FileName = "powershell.exe"
StartInfo.Arguments = $"-ExecutionPolicy Bypass -File ""{ScriptPath}"" " & $"{Device}"""
StartInfo.UseShellExecute = False
StartInfo.RedirectStandardOutput = True
StartInfo.CreateNoWindow = True
Dim MyProcess As Process = Process.Start(StartInfo)
Dim OutData As String = MyProcess.StandardOutput.ReadToEnd()
MyProcess.WaitForExit()
If MyProcess.ExitCode = 0 Then
Dim Separator() As Char = {vbCr, vbLf}
DataLines = OutData.Split(Separator, StringSplitOptions.RemoveEmptyEntries)
Return True
Else
Return False
End If
End Function
Creating a ProcessStartInfo object, I noticed one very useful property, namely the RedirectStandardOutput Boolean which when set to True redirects output using the .StandardOutput.ReadToEnd() method.
So anything sent to the screen goes into an String variable which I can process to eradicate any shitty spaces and bullshit characters ending up with just a couple of strings, namely $FreeSpaceGB and $DriveSizeGB.
In Powershell, if you just enter variables in your script then they are sent to the console. I read this is common practice and after using some other frankly other crappy method, I was delighted at discovering this.
param([string]$RemoteComputer)
$DriveLetter = "C:"
$Session = New-PSSession -ComputerName $RemoteComputer
$DriveInfo = Invoke-Command -Session $session -ScriptBlock { Get-WmiObject -Class Win32_LogicalDisk -Filter "DeviceID='$using:DriveLetter'" }
Remove-PSSession -Session $Session
$DriveSizeGB = [math]::Round($DriveInfo.Size / 1GB, 2)
$FreeSpaceGB = [math]::Round($DriveInfo.FreeSpace / 1GB, 2)
$FreeSpaceGB
$DriveSizeGB
The GetDriveSpace.ps1 script accepts a parameter of the remote computer, gets the space data, writes it to a custom class, and sends it back to the main VB.Net form where I can intercept it and stick those values next to that icon I have painstakingly been trying to ripoff!
I noted the wording on the explorer icon. The GB free is always to two decimal points. This is taken care of in the Powerscript here:
Sub ShowSpaceStatistics()
' Makes the Drivespace, Drivename labels and Drivespace Progress bar, visible.
' Calculates the Percentage of drive space left, and changes the colour to red if lower than 10% free space
' Displays the Drive logo and data to the user
frm_main.pic_remotedrive.Visible = True
frm_main.pic_remotedrive.Image = My.Resources.remotedrive_48x_48x
frm_main.lbl_drivename.Visible = True
frm_main.lbl_drivespace.Visible = True
frm_main.prg_drivespace.Visible = True
frm_main.prg_drivespace.Maximum = DataLines(1)
frm_main.lbl_drivename.Text = "c$ (\\" & RemoteDevice & ") (IPC$)"
frm_main.lbl_drivespace.Text = DataLines(0) & " GB free of " & DataLines(1) & " GB"
Dim Percentage As Double = (DataLines(0) / DataLines(1)) * 100
If Percentage < 10 Then
frm_main.prg_drivespace.ForeColor = Color.Red
Else
frm_main.prg_drivespace.ForeColor = Color.DodgerBlue
End If
Dim Spaceleft As Double = Math.Round(DataLines(1) - DataLines(0), 2)
frm_main.prg_drivespace.Value = Spaceleft
frm_main.prg_drivespace.Maximum = DataLines(1)
End Sub
Then there's the colour of the progress bar that needs to turn RED when the drive is low on space. Googling this again, I found it to be under 10% free and it's no longer BLUE. Not an issue and is addressed here.
If Percentage < 10 Then
frm_main.prg_drivespace.ForeColor = Color.Red
Else
frm_main.prg_drivespace.ForeColor = Color.DodgerBlue
End If
Finally, I needed the HDD space-free value, which is simply a calculation of the total space – the used space, again to two decimal points.
Dim Spaceleft As Double = Math.Round(DataLines(1) - DataLines(0), 2)
Something as simple as recreating the HDD icon with true statistics takes some effort, and though hardly difficult for me, it needed to some tenacity to track down everything I needed.
Oh, and if you believed me about all that Venereal Disease bullshit, then what can I say? My application CURRENTLY only helps people with Trichomoniasis, and I'm adding 'The Clap' support next week.
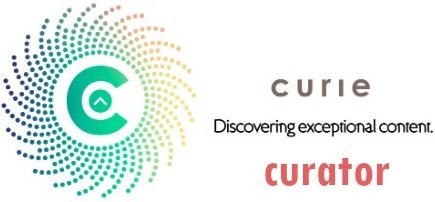
I am always fascinated when someone is able to master coding!
Thanks, but I am hardly a master. A real coder would rip into what I do and take it apart. I can make things work, and even look good but the engine behind it all is barely oiled.
Haha as long as it runs :D I am happy I understand html a little bit 😂 Always wanted to learn coding. But you know…
I have done some of that with CSS lately, integrated with a Python back end and Flask. It's a fucking pain in the arse, like learning to play the keyboard with both hands when you are only used to the high keys. Another of my pet projects!
Haha thats awesome. Whats your favorite coding language?
I don't think I have a favourite. For HIVE development it's always Python, which I only really learned last year. VB.NET I have been using for donkeys years and Powershell I only started getting to grips with properly this year.
To be honest, if you know how to code you can use any of them, its the syntax and idiosyncrasies that trip you up when moving on to another.
I see. And you learned it all by yourself or died you studied it?
It's all self-taught complete with lots of bad habits.
This is awesome! Which ones? If i am allowed to ask :D
All the ones I mentioned, and in the past VBS and KIX.
I am not sure what VBS or KIX is :D
!Pizza
Hehe😂😂
I actually just started learning web development a few weeks ago( it's better late than never lol)...well, so far web development isn't as boring as I thought it was though it can be really tricky at times.
Making code very readable like this takes lots of time and effort too...Nice write up!!
It's not if you understand it and are creating something unique. That's the thing you see, what you do is yours and there's nothing else like it.
Yep very true 👍
Haaaa haaaa haaaa :))... Impossible not to piss laughing reading this, my dear friend and top author!...
!discovery 40
!VSC
!PIZZA
@jlinaresp has sent VSC to @slobberchops
This post was rewarded with 0.1 VSC to support your work.
Join our photography communityVisual Shots
Check here to view or trade VSC Tokens
Be part of our Curation Trail
@jlinaresp ha enviado VSC a @slobberchops
Éste post fue recompensado con 0.1 VSC para apoyar tu trabajo.
Únete a nuestra comunidad de fotografía Visual Shots
Consulte aquí para ver o intercambiar VSC Tokens
Se parte de nuestro Trail de Curación
What you mean.., this is a deadly serious article! 😃
This post was shared and voted inside the discord by the curators team of discovery-it
Join our Community and follow our Curation Trail
Discovery-it is also a Witness, vote for us here
Delegate to us for passive income. Check our 80% fee-back Program
$PIZZA slices delivered:
@jlinaresp(6/10) tipped @slobberchops
Color? Are you turning into a Yank? Mind you, I'm getting influenced after spending some time there.
I've not got to grips with what Power shell can do. The command line stuff seems overcomplicated, but I expect it's powerful. I guess I could do an online course to learn more.
Fixed, all those mandatory 'Color' spelling properties have an effect on the rest of it.
It's a strange language, especially concerning functions and what it returns. You expect a string and end up with a system object!
Where I work, it's heavily used by everyone surrounding me. I go with the flow and it's always had a lot of support from Microsoft with CMDlets to access SCCM (which is what my apps does).
You mad developer bastard, how dare you come here with your dodger blue this and your decimal place that!! 🤣
I thought you might home in on 'The Clap'. I have to find ways to make this a little more readable for the non geeks. 😀
Lol, I was going to but try not to be too predictable :OD
I just found out my niece has to take a Python class and she is freaking out. It has nothing to do with her major and she doesn't have a lot of confidence. I wish I knew more about coding so I could help her. Unfortunately, I have forgotten more than I remember.
I have tried to teach people how to code in the past, you either have the mindset or you don't. It's like teaching me how to draw, it's never going to look good. I don't have the 'artist' flair.
I've done it in the past, so I don't think I am totally inept, I just feel like I am very out of practice.
Coding is not something easy so when I see someone who can do it, I always hail them...
Keep it up!
As a senior developer, my brain hurts not seeing more functions. But as a human I enjoyed your post a lot 😁
You are the type I would love to talk to about my 'style'. What would you do differently given the parts you see? I have never been formally taught to do any of this and I know it's not structured correctly.
Well per example, The most important lesson learned in programming over the years is that variables and function naming is of utmost importance. If you can't understand it 1 year from now, it's not a good name. It's ever more important when you work on code as a team.
OK noted. I am always up for learning from someone who does this all the time (well.., I kind of do that too).
Sure! if you have questions ask away.
Have a good day
Didn't knew you are also into this technical stuff
I'm here for the venereal disease.
Also, fuck Windows.
Now we're talking!
INB4 VD. What all languages do you use man?
Right now, Python, VB.Net and Powershell. I am trying to get to grips with Flask.., but that's simply a python library which is forcing me into HTML and CSS.
I don't know VB, but I do C# & etc. Anyway nice to see this!
$WINE
Wish I knew C#, I use a dated language out of pure laziness.
I want to learn this stuff too. This topic fascinates and entertains me. I also like the way you present programming in this article. Keep up the great work 👍 !PGM
BUY AND STAKE THE PGM TO SEND A LOT OF TOKENS!
The tokens that the command sends are: 0.1 PGM-0.1 LVL-0.1 THGAMING-0.05 DEC-15 SBT-1 STARBITS-[0.00000001 BTC (SWAP.BTC) only if you have 2500 PGM in stake or more ]
5000 PGM IN STAKE = 2x rewards!
Discord
Support the curation account @ pgm-curator with a delegation 10 HP - 50 HP - 100 HP - 500 HP - 1000 HP
Get potential votes from @ pgm-curator by paying in PGM, here is a guide
I'm a bot, if you want a hand ask @ zottone444
I heard that sometimes ChatGPT is giving misleading answers, so even when its answer is seemingly/apparently good, it can still be bad/incorrect. This time it is both.
This time the Segoe UI is indeed the right answer. Segoe UI is the Windows System font. The standard font size has been increased to 9 point, so the 9pt is also right.
But the "they do not disclose those facts" part is not correct in the answer, because there is an article about it on Microsoft Learn: Fonts - Win32 apps - Microsoft Learn.
Yes, it 'lies' a lot, but is also a help. I would rather have it around that not.
Thanks, useful.
😃 the caution almost scared me from reading the post.
It's innovative. I like it. Good work
Nice work i dont know nothing about VB but it is llok awesome :D
Will really take my programming classes seriously now hahaha. I'm done with R and Python already but I'm still not good to it.
I've done a lot of self study into HTML/CSS/JavaScript. Even built some pretty hefty game map logic for some thoughts I've had in my head. However, I find it hard to concentrate on anything when I don't have a secluded lair... My current lair is too subjected to the household racket. I see that Python has quickly taken over as the script language of serving content on the web. I will have to put it on a bucket list of things to do if I ever get a proper coding lair.
Javascript is another one I need to pick up at some point. It looks easy enough to transition into. You can only learn so many, there's too much out there in todays world.
Just do not go handling any vibrant purple dildos
I hope you didn't, I kept my distance. With the way that thing moves around, it's kind of alive!
yeuch!
I still havent been preston, will get round to it in a few weeks its a 4 hour round trip!
You could also try the 'Old Dog Inn', but beware the floors. Not a lot else in that area.
👍
Oh I miss VB. These days I don't do desktop and windows based development. But a lot of desktop based code went through back in time. I also spent a lot of time coding with autohotkey for those shortcut based short apps.
It's very unfashionable in todays world and badly supported in terms of object manipulation. Why is C# the way to go when the code optimization is so similar? I don't get it.
I absolutely 100% don't have the mindset 😂
It's very satisfying knowing you can do this stuff.
I've been trying to get my head around the world of PowerAutomate and PowerBI with M, DAX and Powerfx.
....still shit.
Wow, it has been a while when I wrote code. Nowadays my colleques write a lot of Powershell modules. Next month we will migrate to Powershell 7. Code on !! :)